Before iOS7, developers could either get the system font in regular, italic or bold and a small font, using APIs like this:
static UIFont SystemFontOfSize (float size); static UIFont BoldSystemFontOfSize (float size);
Developers could use the SystemFontSize to get the system font size, but beyond this single number there was no guidance as to what font sizes to use. It was every-man-for-himself kind of place, not too different from the wild west, where people like me would pick 14 because we need glasses, and avant garde designers would pick 11 points.
It was madness!
Guidance
To assist making applications more consistent, developers now have acces a series of pre-configured fonts that can be used in various scenarios, these are available first as properties directly on UIFont:
static UIFont UIFont.PreferredHeadline { get; } static UIFont UIFont.PreferredSubheadline { get; } static UIFont UIFont.PreferredBody { get; } static UIFont UIFont.PreferredCaption1 { get; } static UIFont UIFont.PreferredCaption2 { get; } static UIFont UIFont.PreferredFootnote { get; }
Apple's documentation states that the fonts returned have their properties adjusted to be optimized for reading. The most noticeable attribute those fonts have is the default font and their font sizes.
This is how they look like at the default size:
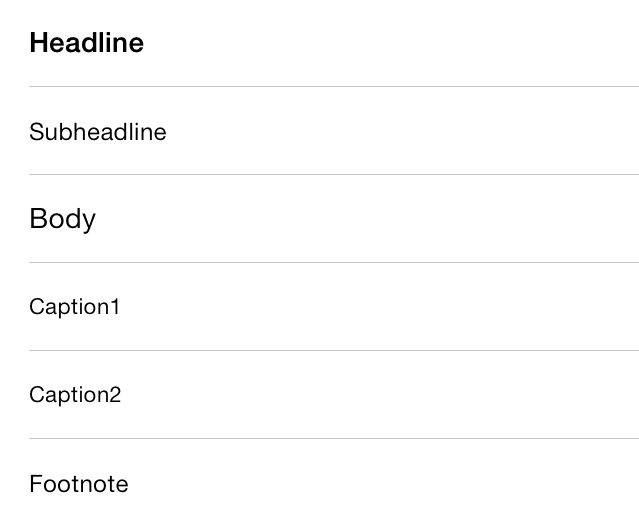
And this is how they look when the text size in the system is set to the largest size (my default):
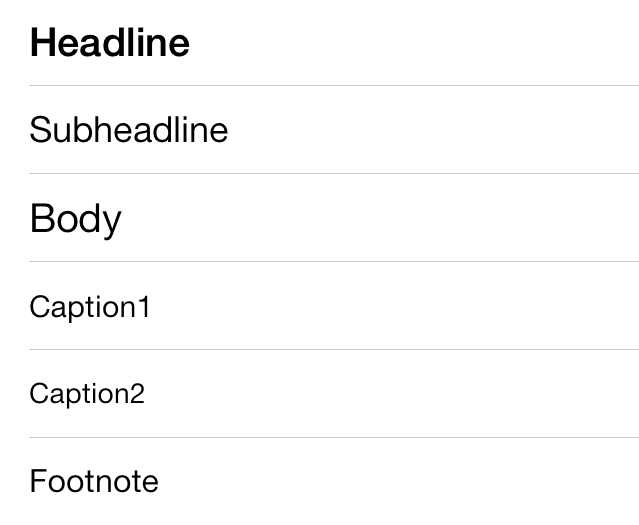
Merely accessing the UIFont
properties will return the
properly sized font depending on the user settings.
Responding to User's settings
With iOS7, the user can change the desired size for his
fonts in the Settings application. Applications in turns
should respond by adjusting their contents. They do this by
using the freshly minted fonts that the system has created for
them in the UIFont
properties and respond to the system
notification that the user has made a font size change.
To do this, you register a UIApplication.Notification, like this:
UIApplication.Notifications.ObserveContentSizeCategoryChanged (delegate {
// text size has changed, reconfigure any UIViews's font here
// Simple:
exampleLabel.font = UIFont.PreferredBody;
});
Customizing the Fonts
UIKit Developers now have access to all of the advanced
typographic control available in CoreText. The interface to
customize fonts is provided by
the UIFontDescriptor
class. You can fetch a
UIFontDescriptor
a UIFont
by accessing its FontDescriptor
property, and you can in turn create UIFonts by configuring a
UIFontDescriptor
and requesting that a font be
created for you.
This is what you do to create a bold or italic fonts:
UIFont MakeBold (UIFont font)
{
var desc = font.FontDescriptor;
// create a new descriptor based on the baseline, with
// the requested Bold trait
var bold = desc.CreateWithTraits (UIFontDescriptorSymbolicTraits.Bold);
// Create font from the bold descriptor
return UIFont.FromDescriptor (bold);
}
You use UIFontDescriptorSymbolicTraits.Italic
for creating an italic font, this is what text looks like for
the PreferredBody by default:
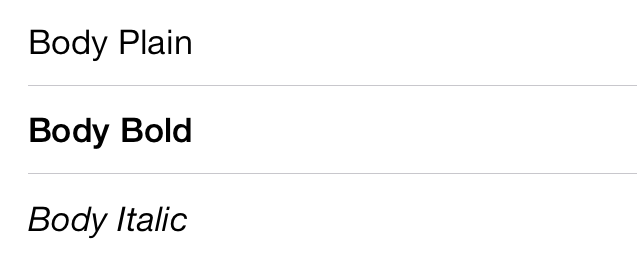
Other simple traits that you can apply to a font include the interline spacing. Something that was not possible previously:
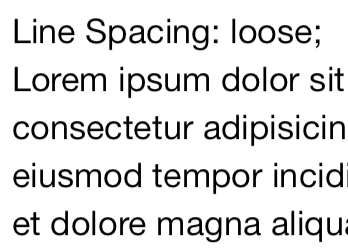
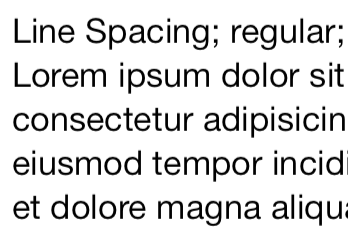
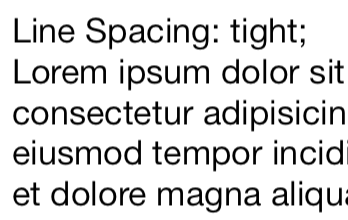
To achieve the above, all you have to do is pass the
UIFontDescriptorSymbolicTraits.TightLeading
or
UIFontDescriptorSymbolicTraits.LooseLeading
parameters to the CreateWithTraits
method.
Using CoreText Font Features
While the above will be enough for many applications, CoreText provides comprehensive access to many features of underlying fonts.
You can use
the UIFontDescriptor.CreateWithAttributes
method to pass your detailed list
of UIFontAttributes
that you want to set on your font.
The following example shows how to request the use of proportional numbers in the default font:
public UIFont ResizeProportional (UIFont font)
{
var attributes = new UIFontAttributes (
new UIFontFeature (
CTFontFeatureNumberSpacing.Selector.ProportionalNumbers));
var newDesc = font.FontDescriptor.CreateWithAttributes (attributes);
return UIFont.FromDescriptor (newDesc, 40);
}
The nice thing about these strong types is that code-completion in the editor basically provides you with a visual guide of which attributes are available for you to use. This is the result of the above code when rendering numbers:
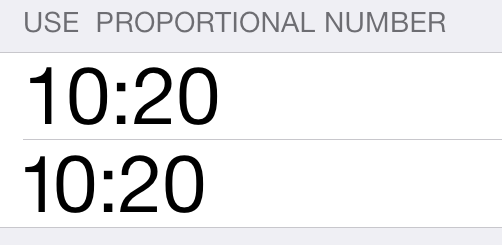
The above is just a sample, you can use any of the CoreText Font Features to customize the rendering of your font.
Some other variations:
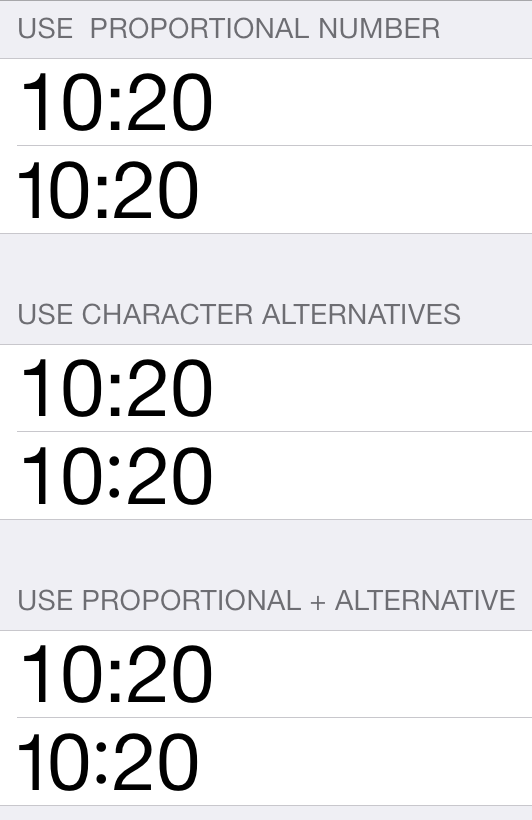
The entire sample showing these features is available in the ios7fonts directory.