Starting with iOS 5 it is possible to more easily style your UIViews. Apple did this by exposing a new set of properties on most views that can be tuned. For example, to configure the TintColor of a UISlider, you would write:
var mySlider = new UISlider (rect); mySlider.ThumbTintColor = UIColor.Red;
You can also set the color globally for all instances of UISlider, you do this by assigning the styling attributes on the special property "Appearance" from the class you want to style.
The following example shows how to set the Tint color for all UISliders:
UISlider.Appearance.ThumbTintColor = UIColor.Red;
It is of course possible to set this on a per-view way,
The first time that you access the "Appearance" static property on a stylable class a UIAppearance proxy will be created to host the style changes that you have requested and will apply to your views.
In Objective-C the Appearance property is untyped. With MonoTouch we took a different approach, we created a strongly typed UIXxxxAppearance class for each class that supports styling. Our generated UIXxxxxAppearance class is strongly typed, which allows users to use intellisense to easily discover which properties are avaialble for styling.
We also created a hierarchy that reflects the inherited appearance properties, this is the class hierarchy for the UISLider.UISliderAppearance class:
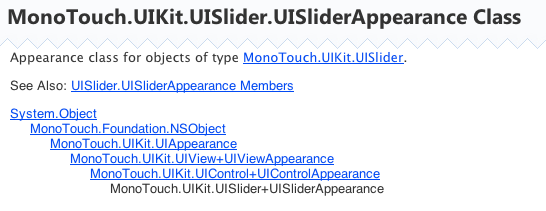
The properties exposed by UISlider for example are:
public class UISliderAppearance { public virtual UIColor BackgroundColor { get; set; } public virtual UIColor MaximumTrackTintColor { get; set; } public virtual UIColor MinimumTrackTintColor { get; set; } public virtual UIColor ThumbTintColor { get; set; } }
MonoTouch also introduced support for styling your controls only when they are hosted in a particular part of the hierarchy. You do this by calling the static method AppearanceWhenContainedIn which takes a variable list of types, it works like this:
var style = UISlider.AppearanceWhenContainedIn (typeof (SalesPane), typeof (ProductDetail)); style.ThumbTintColor = UIColor.Red;
In the above sample the style for the ThumbTintColor will be red, but only for the UISliders contained in ProductDetail view controllers when those view controllers are being hosted by a SalesPane view controller. Other UISliders will not be affected.
Both the Appearance static property and the AppearanceWhenContainedIn static method have been surfaced on every UIView that supports configuring its style. Both of them return strongly typed classes that derive from UIAppearance and expose the exact set of properties that can be set.
This is different from the weakly typed Objective-C API which makes it hard to discover what can be styled.