This morning Andres came by IRC asking questions about Key Value Observing, and I could not point him to a blog post that would discuss the details on how to use this on C#.
Apple's Key-Value Observing document contains the basics on how to observe changes in properties done to objects.
To implement Key-Value-Observing using MonoTouch or MonoMac all you have to do is pick the object that you want to observe properties on, and invoke the "AddObserver" method.
This method takes a couple of parameters: an object that will be notified of the changes, the key-path to the property that you want to observe, the observing options and a context object (optional).
For example, to observe changes to the "bounds" property on a UIView, you can use this code:
view.AddObserver ( observer: this, keyPath: new NSString ("bounds"), options: NSKeyValueObservingOptions.New, context: IntPtr.Zero);
In this example, I am using the C# syntax that uses the Objective-C style to highlight what we are doing, but you could just have written this as:
view.AddObserver ( this, new NSString ("bounds"), NSKeyValueObservingOptions.New, IntPtr.Zero);
What the above code does is to add an observer on the "view" object, and instructs it to notify this object when the "bounds" property changes.
To receive notifications, you need to override the ObserveValue method in your class:
public override void ObserveValue (NSString keyPath, NSObject ofObject, NSDictionary change, IntPtr context) { var str = String.Format ( "The {0} property on {1}, the change is: {2}", keyPath, ofObject, change.Description); label.Text = str; label.Frame = ComputeLabelRect (); }
This is what the app shows if you rotate your phone:
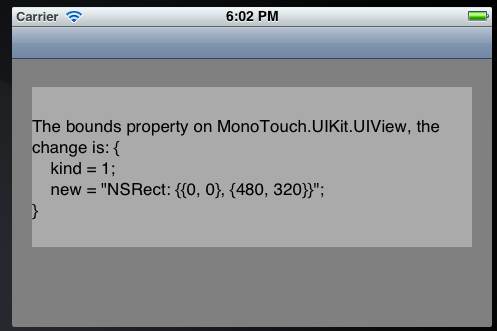
The complete sample has been uploaded to GitHub.